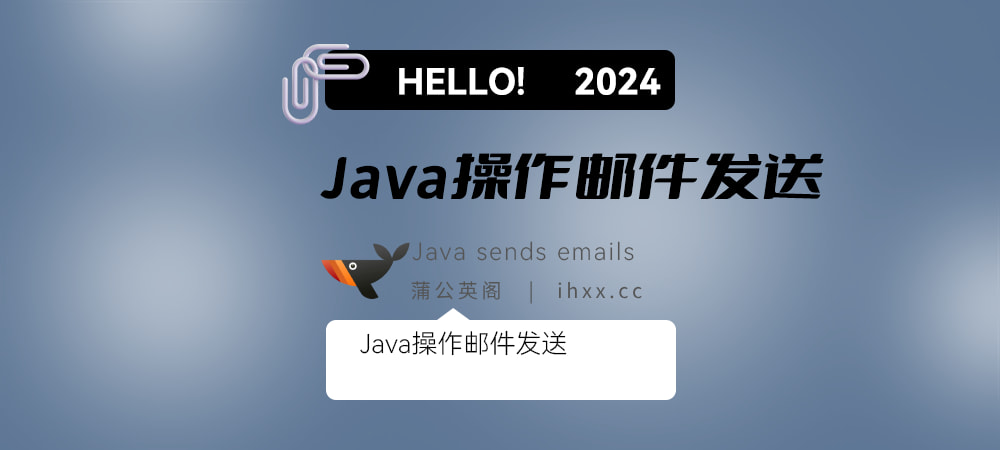
Java操作邮件发送
AI-摘要
切换
鸡哔你 GPT
AI初始化中...
介绍自己
生成本文简介
推荐相关文章
前往主页
前往tianli博客
Java操作邮件发送
java发送Email,不限制邮件类型,邮箱号和私钥获取来就行
pom依赖
<!-- 邮件 spring-boot-starter-mail -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
<version>2.3.4.RELEASE</version>
</dependency>
<!-- thymeleaf模板 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
<version>2.2.13.RELEASE</version>
</dependency>
mail 发送邮件
thymeleaf 模板解析
实现
不配合项目配置文件使用的
package com.xx.xx.xx.config.email;
import com.sun.mail.util.MailSSLSocketFactory;
import freemarker.template.Configuration;
import freemarker.template.Template;
import lombok.extern.slf4j.Slf4j;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.ui.freemarker.FreeMarkerTemplateUtils;
import org.springframework.web.servlet.view.freemarker.FreeMarkerConfigurer;
import javax.mail.Address;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.security.GeneralSecurityException;
import java.util.*;
/**
* <p>
*
* </p>
*
* @author lph
* @package com.xx.xx.xx.config.email
* @project xx
* @since 2022 -05 -25 11:49
*/
@Slf4j
public class EmailConfig {
public static final String DEFAULT_EMAIL = "我设置的默认收件人";
public static void sendEmail(Map<String, Object> params) {
try {
//我将模板内解析信息 也放在了map中
String ACCOUNT=params.get("account").toString();
String PWD =params.get("pwd").toString();
String SMTPSERVER =params.get("server").toString();
// 创建邮件配置 这些配置是不需要改动的
Properties props = new Properties();
props.setProperty("mail.transport.protocol", "smtp");
props.setProperty("mail.smtp.host", params.get("server").toString());
props.setProperty("mail.smtp.auth", "true");
props.setProperty("mail.smtp.timeout", "994");
props.setProperty("mail.smtp.port", "465");
MailSSLSocketFactory sf = new MailSSLSocketFactory();
sf.setTrustAllHosts(true);
props.put("mail.smtp.ssl.enable", "true");
props.put("mail.smtp.ssl.socketFactory", sf);
props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
props.setProperty("mail.smtp.auth", "true");
props.setProperty("mail.smtp.ssl.enable", "true");
Session session = Session.getDefaultInstance(props);
FreeMarkerConfigurer freeMarkerConfigurer = new FreeMarkerConfigurer();
Configuration cfg = new Configuration(Configuration.VERSION_2_3_27);
cfg.setClassLoaderForTemplateLoading(ClassLoader.getSystemClassLoader(), "template");
freeMarkerConfigurer.setConfiguration(cfg);
Template template = freeMarkerConfigurer.getConfiguration().getTemplate("EmailTemplate.ftl");
String mailText = FreeMarkerTemplateUtils.processTemplateIntoString(template, params);
MimeMessage message = createEmail(session, mailText, params);
log.info("邮件信息创建完毕!");
Transport transport = session.getTransport();
transport.connect(SMTPSERVER, ACCOUNT, PWD);
transport.sendMessage(message, message.getAllRecipients());
log.info("邮件发送成功!");
transport.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public static MimeMessage createEmail(Session session, String context, Map<String, Object> params) throws Exception {
MimeMessage msg = new MimeMessage(session);
MimeMessageHelper messageHelper = new MimeMessageHelper(msg, true, "utf-8");
InternetAddress fromAddress = new InternetAddress(params.get("account").toString(),
params.get("title").toString(), "utf-8");
msg.setFrom(fromAddress);
Address[] internetAddressTo = InternetAddress.parse(params.get("users").toString());
msg.setRecipients(MimeMessage.RecipientType.TO, internetAddressTo);
msg.setSubject("标题", "utf-8");
messageHelper.setText(context, true);
msg.setSentDate(new Date());
msg.saveChanges();
return msg;
}
}
//业务内调用
Map<String, Object> params = new HashMap<>(18);
params.put("users", "收件人列表 逗号隔开");
params.put("server","服务地址");
params.put("account","发件人账号");
params.put("pwd","私钥"));
EmailConfig.sendEmail(params);
模板 放在resource下 自行查找即可
数据库
CREATE TABLE `tb_send_email_conf`
(
`email_id` int(11) NOT NULL AUTO_INCREMENT COMMENT 'email配置id',
`email_account` varchar(30) DEFAULT NULL COMMENT '邮件账号',
`email_type` tinyint(1) NOT NULL COMMENT '1-发送方 2-接收方 3-即是发送方又是接收方',
`email_server` varchar(30) DEFAULT NULL COMMENT '发送方邮件账号的服务地址',
`email_pwd` varchar(255) DEFAULT NULL COMMENT '发送方邮件的秘钥',
`enable_email` tinyint(1) unsigned zerofill DEFAULT '0' COMMENT '开启发送和接收 0-关闭 1-开启',
`remarks` varchar(200) CHARACTER SET utf8 DEFAULT NULL COMMENT '备注',
`create_user` int(11) DEFAULT NULL COMMENT '创建人ID',
`create_time` timestamp NULL DEFAULT NULL ON UPDATE CURRENT_TIMESTAMP COMMENT '创建日期',
`update_user` int(11) DEFAULT NULL COMMENT '编辑人ID',
`update_time` timestamp NULL DEFAULT NULL COMMENT '编辑日期',
`deleted_flag` tinyint(1) NOT NULL DEFAULT '0' COMMENT '删除状态(0正常,1逻辑删除)',
PRIMARY KEY (`email_id`)
) ENGINE = InnoDB
AUTO_INCREMENT = 1
DEFAULT CHARSET = utf8mb4 COMMENT ='邮件配置';
使用配置文件的
package com.xx.xx.xx.config.email;
import com.sun.mail.util.MailSSLSocketFactory;
import freemarker.template.Configuration;
import freemarker.template.Template;
import lombok.extern.slf4j.Slf4j;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.ui.freemarker.FreeMarkerTemplateUtils;
import org.springframework.web.servlet.view.freemarker.FreeMarkerConfigurer;
import javax.mail.Address;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.security.GeneralSecurityException;
import java.util.*;
/**
* <p>
*
* </p>
*
* @author lph
* @package com.xx.xx.xx.config.email
* @project xx
* @since 2022 -05 -25 11:49
*/
@Slf4j
public class EmailConfig {
/**
* 获取属性配置
*/
private static Properties properties = new Properties();
public static final String DEFAULT_EMAIL = "默认收件人";
/**
* 静态块初始化配置
*/
static {
try {
properties.setProperty("mail.smtp.auth", "true");
properties.setProperty("mail.smtp.timeout", "994");
properties.setProperty("mail.smtp.server", "smtp.qq.com");
properties.setProperty("mail.smtp.port", "465");
properties.setProperty("mail.smtp.account", "发件人");
properties.setProperty("mail.smtp.pwd", "秘钥");
MailSSLSocketFactory sf = new MailSSLSocketFactory();
sf.setTrustAllHosts(true);
properties.put("mail.smtp.ssl.enable", "true");
properties.put("mail.smtp.ssl.socketFactory", sf);
} catch (GeneralSecurityException e) {
e.printStackTrace();
}
}
/**
* 从属性文件中获取值其中key为smtp.server
*/
public static String SMTPSERVER = properties.getProperty("mail.smtp.server");
/**
* 端口号
*/
public static String SMTPPORT = properties.getProperty("mail.smtp.port");
/**
* 账户名
*/
public static String ACCOUT = properties.getProperty("mail.smtp.account");
/**
* 授权密码
*/
public static String PWD = properties.getProperty("mail.smtp.pwd");
public static void sendEmail(Map<String, Object> params) {
try {
// 创建邮件配置
Properties props = new Properties();
props.setProperty("mail.transport.protocol", "smtp");
props.setProperty("mail.smtp.host", SMTPSERVER);
props.setProperty("mail.smtp.port", SMTPPORT);
props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
props.setProperty("mail.smtp.auth", "true");
props.setProperty("mail.smtp.ssl.enable", "true");
Session session = Session.getDefaultInstance(props);
FreeMarkerConfigurer freeMarkerConfigurer = new FreeMarkerConfigurer();
Configuration cfg = new Configuration(Configuration.VERSION_2_3_27);
cfg.setClassLoaderForTemplateLoading(ClassLoader.getSystemClassLoader(), "template");
freeMarkerConfigurer.setConfiguration(cfg);
Template template = freeMarkerConfigurer.getConfiguration().getTemplate("EmailTemplate.ftl");
String mailText = FreeMarkerTemplateUtils.processTemplateIntoString(template, params);
params.put("title", "模板内数据");
MimeMessage message = createEmail(session, mailText, params);
log.info("邮件信息创建完毕!");
Transport transport = session.getTransport();
transport.connect(SMTPSERVER, ACCOUT, PWD);
transport.sendMessage(message, message.getAllRecipients());
log.info("邮件发送成功!");
transport.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public static MimeMessage createEmail(Session session, String context, Map<String, Object> params) throws Exception {
MimeMessage msg = new MimeMessage(session);
MimeMessageHelper messageHelper = new MimeMessageHelper(msg, true, "utf-8");
InternetAddress fromAddress = new InternetAddress(ACCOUT,
params.get("title").toString(), "utf-8");
msg.setFrom(fromAddress);
Address[] internetAddressTo = InternetAddress.parse(params.get("users").toString());
msg.setRecipients(MimeMessage.RecipientType.TO, internetAddressTo);
msg.setSubject("邮件标题", "utf-8");
messageHelper.setText(context, true);
msg.setSentDate(new Date());
msg.saveChanges();
return msg;
}
}
本文是原创文章,采用CC BY-NC-ND4.0协议,完整转载请注明来自蒲公英阁
评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果